// Again one more switch condition statement....
using System;
namespace symbols
{
class class1
{
static void Main()
{
char ch;
int inum1,inum2,inum3;
double dnum;
Console.WriteLine("Enter first nmber");
inum1=Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter second nmber");
inum2=Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter your choice + , - , * or /");
ch=Convert.ToChar(Console.ReadLine());
switch(ch)
{
case '+' :
inum3=inum1+inum2;
Console.WriteLine("Sum is "+inum3);
break;
case '-' :
inum3=inum1-inum2;
Console.WriteLine("Difference is "+inum3);
break;
case '*' :
inum3=inum1*inum2;
Console.WriteLine("Product is "+inum3);
break;
case '/' :
dnum=(Double)(inum2/inum1);
Console.WriteLine(" Quotient is "+dnum.ToString());
break;
default :Console.WriteLine("entered a wrong choice");
break;
}
}
}
}
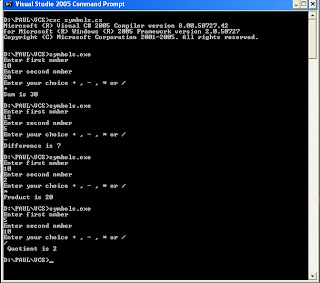