using System;
Search........
Friday, March 7, 2008
While Loop
using System;
Looping Statements
inum1=Convert.ToInt32(Console.ReadLine());
inum2=Convert.ToInt32(Console.ReadLine());
inum3=Convert.ToInt32(Console.ReadLine());
inum4=Convert.ToInt32(Console.ReadLine());
inum5=Convert.ToInt32(Console.ReadLine());
inum6=Convert.ToInt32(Console.ReadLine());
.
.
.
.
inum100=Convert.ToInt32(Console.ReadLine());
so to over come these problem we are using the loop sattements....Here we will use a loop statement the body will be executed until the condition turns false
Arithmetic operators with switch
using System;
switch statements
syntax :- switch(expression)
{
case value1:
body;
break;
case value2:
body;
break;
case value3:
body;
break;
default :
body;
break; //executes if all other conditions are wrong....
*/
using System;
namespace switchdemo
{
class class1
{
static void Main()
{
char ch;
Console.WriteLine("enter your choice (a,b,c,d)");
ch=Convert.ToChar(Console.ReadLine());
switch(ch)
{
case 'a' :
Console.WriteLine("This is from case a");
break;
case 'b' :
Console.WriteLine("This is from case b");
break;
case 'c' :
Console.WriteLine("This is from case c")
;
break;
case 'd' :
Console.WriteLine("This is from case d");
break;
default :
Console.WriteLine("This not from the specified choice");
break;
}
}
}
}
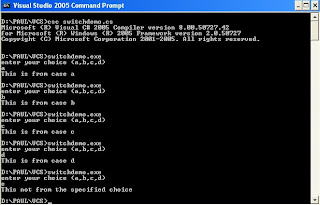
Conditional Statements
*/
using System;
Double...
using System;
Thursday, March 6, 2008
converting to character
/ /converting to character .......
using System;
namespace nm1
{
class cl1
{
static void Main()
{
char ch;
Console.WriteLine("enter a character");
ch=Convert.ToChar(Console.ReadLine());
Console.WriteLine("The character entered is "+ ch);
}
}
}
/* Here we are going to add two numbers */
using System;
namespace nm1
{
class cl1
{
static void Main()
{
int inum1,inum2,add;
Console.WriteLine("enter a no");
inum1=Convert.ToInt32(Console.ReadLine());
Console.WriteLine("enter a second no.");
inum2=Convert.ToInt32(Console.ReadLine());
add=inum1+inum2;
Console.WriteLine("Result ="+add.ToString());
}
}
convert To Int
Lets try converting the program from string to int,float ,double,char
To interger "Convert.ToInt32(inputed value)";
To float we use "float.Parse(inputed value)",
To double "Convert.ToDouble(inputed value)",
To Character "Convert.ToChar(inputed value)"
using System;
{
class cls1
{
static void Main()
{
String str;
int inum;
Console.WriteLine(" Enter a number");
str=Console.ReadLine();
inum=Convert.ToInt32(str); // Here we are converting
Console.WriteLine("The numbe entred is "+inum);
}
}
}

Wednesday, March 5, 2008
Input
using System;
namespace inputdemo
{
class cls1
{
static void Main()
{
String str;
Console.WriteLine(" Enter Your Name");
str=Console.ReadLine();
Console.WriteLine("The Name Entered is "+str);
}
}
}

Sunday, March 2, 2008
How we will get the input....
namespace name
{
class Hello
{
static void Main()
{
String str;
Console.WriteLine(" Enter Your Name");
str=Console.ReadLine(); //here we will get the input , it is in string type
Console.WriteLine("Your Name is "+str);
}
}
}
Hello World......
using System;
namespace Hello
{
class world
{
static void Main()
{
Console.WriteLine("Hello World");
}
}
}
Console
Note:-
Save the progarm with the extension .cs (if it is csharp)
eg:-program.cs
To compile the program go to the visual studio and take the visual studio command promt......
Change the control from the present folder to the folder where your program is stored
Suppose ur program is at D:\paul\vcs\program.cs
now to change your contorl use
c:\visuall\v8>d: <-(enter)
d:\>cd paul <-
d:\paul>cd vcs <-
D:\paul\vcs>csc progarm.cs
To run
D:\paul\vcs>program.exe <-
About Dot Net and How it works
The .NET initiative offers a complete suite for developing and deploying applications. The suite consists of
.NET Products
.NET Services and
.NET Framework
The .NET Framework:-
It is the foundation on which you design, develop and deploy applications.
Its consistent and simplified programming model makes it easier to build robust applications.
The .NET Class Framework consists of a class library that works with any .NET language, such as Visual Basic and C#.
This class library is build in Object-oriented nature of the runtime
The .NET frame work base classes:-
The .NET framework base classes consists of Namespaces and Assemblies
Namespaces helps to create logical groups of related classes and interfaces.
An Assembly is a single deployable unit that contains all the information about the implementation of classes, Structures and Interfaces
COMMON LANGUAGE RUNTIME:-
Common Language Runtime provides functionality such as exception handling, security, debugging and versioning support to any language that targets it.
The CLR can execute programs written in any language.
HOW IT EXECUTE:-
When you compile a program developed in a language that targets the CLR, instead of compiling the source code into machine-level code, the compiler translates it into Microsoft Intermediate Language(MSIL) or Intermediate Language(IL). No matter which language has been used to develop the application it always gets translated into IL
The compiler created the .EXE or .DLL file.
When you execute the .EXE or .DLL file, the code and all the other relevant information from the base class library is sent to the class loader.
The Just In Time(JIT) compiler translates the code from IL into managed native code. The CLR supplies a JIT compiler for each supported CPU architecture.
During the process of compilation , the JIT compiler compiles only the code that is required during execution instead of compiling the complete IL code.
During JIT compilation the is also checked for type safety
After translating the IL into native code, the converted code is sent to the .NET runtime manager.
The .NET runtime manager executes the code. While executing the code, a security check is performed to ensure that the code has the appropriate permissions for accessing the available resources
Know About Object Oriented Programming
Object Oriented Programming was first introduced by Dr. E F Codd in 1970’s.
OOP concept is related to concept of Classes and Objects.The main reason for introducing the oops is to provide data security.
Class :-
Class is a user defined Data Type
Class is just a Virtual concept of a collection of related items together which we cannot see or feel.
Object:-
Object is an Instance of a Class. We can access a Class only through its Objects.
A thing which has the properties of a class is known as Objects.
For eg:- Car is a Class and Benz is an Object of the class 'Car'
An Object have it's own identity
e.g:- From many Benz cars we identify it by its registration number
The main characteristics of Object Oriented Programming are:-
- Inheritance
- Encapsulation
- Abstraction
- Polymorphism
Inheritance:-
The ability of a class (Derived Class) to derive or Inherit the properties of another class (Base Class) is called Inheritance
eg:-A child inherits Many Property from his parents But it is not necessary to inherit all the properties....
Encapsulation:-
It is the process of wrapping of data and functions into a single unit
Process of Hiding the data from a user is called Encapsulation; also called Data Hiding.
Abstraction:-
The process of showing essential features without giving any explanations is known as Abstraction.
eg:- In case of a car a Person know only how to drive the car..its gear,break and all that only essintal for driving that car
Polymorphism:-
Poly means 'many
morphism means forms'
It is the process of existing in more than one form...
eg:- Behavior of a person in front of his authority and friends
Here c++ ,c# and java are object oriented programs
Before we start....
Variable is a named memory location
For a variable there will be memory address
value
e.g. :- inum,inum1,fnum,cname;
Rules For Declaring Variables :-
A Variable Should Start with an Alphabet
e.g. a, b,a1,name
Key Words are not allowed
e.g. int,float....etc
No special characters are allowed
e.g. @,#,! Etc... Exception is underscore(_)
Numbers are allowed ....
e.g. a12,b034 etc
Number should not be the Starting
e.g. 12a,13a these are wrong decleration
Data Types :-
Data type shows which type of values should a variable hold
There are mainly four types of basic data types
int - integer
float - floatting
double - double type value
char - character type value
e.g : - int a=10;
float c=15.5;
double aa=10.557857;
char ch=‘a’;
here a is a character not a variable so it is in single codes
Operator :-
Operators are used to do perform some operations on the variables
There are three types of operators
Arithmetic Operators
Relational ,,
Logical ,,
Arithmetic Operators :-
Arithmetic Operators are used to do mathematical operations
e.g . c=a+b;
The basic arithmetic Operators are
int a,b,c;
+ for addition e.g : c= a+b
- for subtraction e.g : c= a-b
* multiplication e.g : c=a*b;
/ division e.g : c=a/b; (5/2=2)
% modulo operator (returns remainder after division)
e.g : c=(a%b); (5%2=1)
Relational Operators:-
It shows the relation between the two variables
e.g : > (greater than)
< (less than)
>=(greater than or equal to)
<= (less than or equal to)
==(equals)
!=(not equal to)
e,g : (a>b),(a=b),(a!=b);
Logical Operators :
&& - Logical AND
e.g.(a>b&&a>c)
|| - Logical OR
e.g.(a>b||a==b)
! - Logical NOT
e.g(!a)